What is Python?
In the realm of programming languages, Python stands as a versatile and widely used choice, renowned for its clear syntax, readability, and beginner-friendliness. This dynamically typed, interpreted language executes code as it is written, eliminating the need for prior compilation. Python’s versatility extends to a diverse range of applications, encompassing web development, data science, machine learning, and artificial intelligence.
Why Python?
Python’s widespread adoption stems from its numerous advantages that make it an ideal choice for a variety of programming tasks:
- Readability and Simplicity: Python’s syntax is concise and easy to comprehend, making it an excellent choice for both novices and experienced programmers alike.
- Versatility: Python’s vast standard library and extensive collection of third-party libraries provide a rich toolkit for various applications, from web development to data analysis and scientific computing.
- Portability: Python’s code seamlessly runs across different operating systems, including Windows, macOS, and Linux, making it a portable and flexible language.
- Interpreted Nature: Python’s interpreted nature allows for rapid prototyping and faster development cycles, enabling quick experimentation and iteration.
- Active Community: Python boasts a large and active community of developers, providing ample support and resources for learning and troubleshooting.
Who Should Learn Python?
Python’s suitability extends to a diverse range of individuals, including:
- Beginners: Python’s beginner-friendly syntax and comprehensive learning resources make it an excellent choice for those new to programming.
- Experienced Programmers: Python’s versatility and extensive libraries offer experienced programmers a powerful tool for various tasks.
- Web Developers: Python’s robust web development frameworks, such as Django and Flask, make it a popular choice for building web applications.
- Data Scientists: Python’s extensive data analysis libraries, such as NumPy, Pandas, and Matplotlib, make it a powerful tool for data manipulation and visualization.
- Machine Learning Enthusiasts: Python’s libraries like scikit-learn and TensorFlow provide a comprehensive toolkit for developing machine learning models.
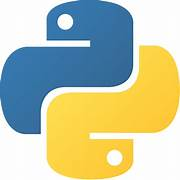
Installation and Setup
Installing Python
- Download Python: Visit the official Python website (https://www.python.org/downloads/) and download the latest stable version of Python for your operating system.
- Execute the Installer: Run the downloaded installer and follow the on-screen instructions to install Python on your system.
- Verify Installation: Open a terminal or command prompt and type
python3 --version
. If Python is installed correctly, it will display the installed version.
Setting up your development environment
- Choose an IDE (Integrated Development Environment): Popular IDEs for Python include PyCharm, Visual Studio Code, and Sublime Text.
- Install a Virtual Environment (Optional): A virtual environment isolates your project’s dependencies from the global Python environment, preventing conflicts.
- Set up a Code Editor: If you prefer a simple code editor, options like Vim, Nano, or Notepad++ are available.
Basic Syntax
Variables and Data Types
Python supports various data types, including integers, floats, strings, and Booleans. Variables are used to store data and can be assigned values using the =
operator.
Sample Code:
Python
# Assigning values to variables
x = 10
y = 3.14
name = "John Doe"
is_active = True
Operators and Expressions
Python provides a range of operators for performing arithmetic, logical, and comparison operations. Expressions combine variables, operators, and values to produce results.
Sample Code:
Python
# Arithmetic operation
sum = x + y
# Logical operation
comparison = x > y
# Comparison operation
is_equal = name == "John Doe"
Control Flow Statements
Control flow statements determine the execution order of code, allowing for conditional branching and looping. Python’s control flow statements include if
, elif
, else
, for
, and while
.
Sample Code:
Python
# Conditional branching
if is_active:
print("User is active")
else:
print("User is inactive")
# Looping
for i in range(1, 10):
print(i)
Functions
Functions encapsulate reusable code blocks, allowing for modularity and code organization. Functions can receive input arguments, perform operations, and return results.
Sample Code:
Python
# Defining a function
def greet(name):